Web Optimized Image Delivery for AEM Custom Components
Published
Viewed136 times
Using Core Components
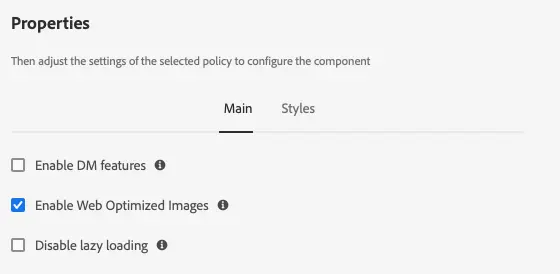
To confirm the changes, you can navigate to "View as Published" by checking the content-type of loaded images in the browser's developer tools network tab.
WebP Image for Custom Components
For Custom Components, AEM provides the
AssetDelivery
Java API, which acts as an OSGi service that generates web optimized delivery URLs for image assets. The AssetDelivery OSGi Service functions in AEM as a Cloud Service but returns null in the AEM SDK. It is best to conditionally use web optimized URLs selectively for Cloud Service and fallback URLs for the SDK.WebOptimizedImageServiceImpl.java
public class WebOptimizedImageServiceImpl implements WebOptimizedImageService {
private static final String DEFAULT_FORMAT = "webp";
private volatile AssetDelivery assetDelivery;
public String getDeliveryURL(ResourceResolver resourceResolver, String path) {
Resource resource = resourceResolver.getResource(path);
Asset asset = DamUtil.resolveToAsset(resource);
if (assetDelivery != null) {
return getWebOptimizedUrl(resource, asset);
} else {
return getFallbackUrl(asset);
}
}
private String getWebOptimizedUrl(Resource resource, Asset asset) {
Map<String, Object> options = new HashMap<>();
options.put("preferwebp", "true");
// These 3 options are required for the AssetDelivery API to work
options.put("path", asset.getPath());
options.put("format", DEFAULT_FORMAT);
options.put("seoname", asset.getName());
return assetDelivery.getDeliveryURL(resource, options);
}
private String getFallbackUrl(Asset asset) {
return asset.getPath();
}
}
The Sling Model uses the custom WebOptimizedImageService OSGi service to obtain the web optimized image URL.
ArticleImpl.java
public class ArticleImpl implements Article {
protected static final String RESOURCE_TYPE = "aem-demo/components/article";
protected ResourceResolver resourceResolver;
private WebOptimizedImageService webOptimizedImageService;
String fileReference;
public String getImageUrl() {
return webOptimizedImageService.getDeliveryURL(resourceResolver, fileReference);
}
}
You can now use the
imageUrl
in your component HTML and inspect the image's content-type through the network tab of the browser's developer tools.