Replicate data-sly-resource in AEM SPA Component
Published
Viewed128 times
data-sly-resource
in a Single Page Application (SPA), we can utilize the Container core component. Here are the steps that need to be followed.- Create a proxy component by extending Container core component.teaser / .content.xml
<jcr:root xmlns:sling="http://sling.apache.org/jcr/sling/1.0" xmlns:cq="http://www.day.com/jcr/cq/1.0" xmlns:jcr="http://www.jcp.org/jcr/1.0" jcr:primaryType="cq:Component" cq:isContainer="{Boolean}true" jcr:title="Teaser" sling:resourceSuperType="core/wcm/components/container/v1/container" componentGroup="AEM React (SPA) - Content"/>
- Included the components you want to embed, which will be added to the JCR as soon as the component is dropped in the parsys.teaser / _cq_template / .content.xml
<jcr:root xmlns:sling="http://sling.apache.org/jcr/sling/1.0" xmlns:cq="http://www.day.com/jcr/cq/1.0" xmlns:jcr="http://www.jcp.org/jcr/1.0" jcr:primaryType="nt:unstructured"> <teaser_text jcr:primaryType="nt:unstructured" sling:resourceType="aem-react-spa/components/text"/> <teaser_action jcr:primaryType="nt:unstructured" sling:resourceType="aem-react-spa/components/button"/> <teaser_image jcr:primaryType="nt:unstructured" sling:resourceType="aem-react-spa/components/image"/> </jcr:root>
- Fetch embedded components in Sling Model using Delegation Pattern.Teaser.java
public class Teaser implements LayoutContainer { protected static final String RESOURCE_TYPE = "aem-react-spa/components/teaser"; private LayoutContainer layoutContainer; public String getExportedType() { return TextImageImpl.RESOURCE_TYPE; } private interface DelegationExclusion { String getExportedType(); } }
- In the last step, add the embedded components into the React Component, where
this.childComponents
containing all embedded elements, aligning with the order specified in the_cq_template
. For instance, index 0 represents text, index 1 represents a button, and so forth.Teaser.tsxclass Teaser extends Container<ContainerProperties, ContainerState> { render() { return ( <div className='cmp-teaser'> <div className='cmp-teaser__text'> {this.childComponents[0]} </div> <div className='cmp-teaser__action'> {this.childComponents[1]} </div> <div className='cmp-teaser__image'> {this.childComponents[2]} </div> </div> ); } }
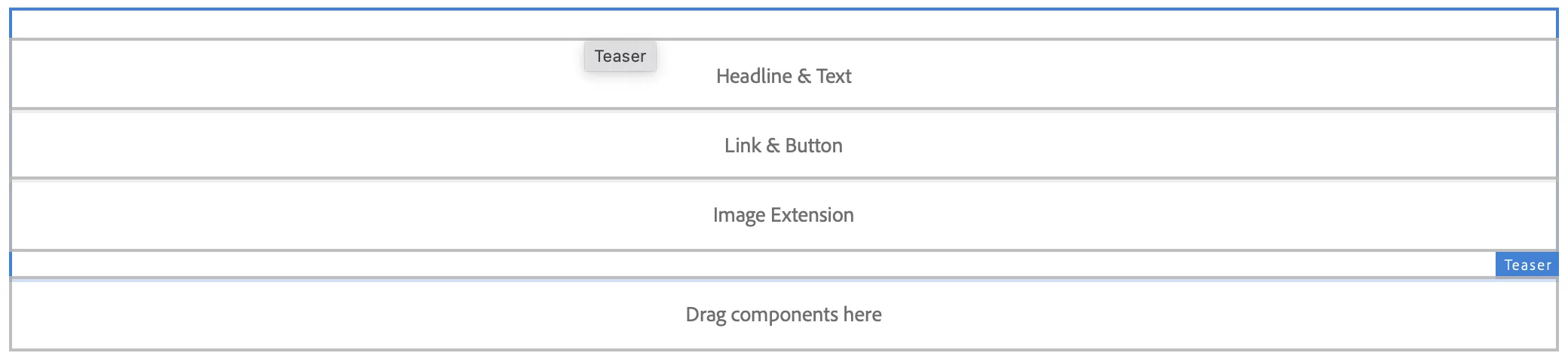