Custom OSGi Configuration in AEM
Published
Viewed108 times
/system/console/configMgr
while custom configurations can be created as per business requirements. These configurations are typically managed within the AEM project's ui.config
module in the code repository.AppConfig.java
public AppConfig {
String app_name();
String api_endpoint();
String client_id();
String client_secret();
}
AppConfigService.java
public interface AppConfigService {
String getAppName();
String getAPIEndpoint();
String getClientIds();
String getClientSecret();
}
AppConfigServiceImpl.java
public class AppConfigServiceImpl implements AppConfigService {
private AppConfig appConfig;
public void activate(AppConfig appConfig) {
this.appConfig = appConfig;
}
public String getAppName() {
return appConfig.app_name();
}
public String getAPIEndpoint() {
return appConfig.api_endpoint();
}
public String getClientId() {
return appConfig.client_id();
}
public String getClientSecret() {
return appConfig.client_secret();
}
}
ui.config / osgiconfig
folder of your project. If the configuration values differ across environments or run modes, you should add separate configurations within the respective environment or run mode-specific folders like config.publish.dev
, config.publish.stage
, etc.config / com.aem.demo.core.services.impl.AppConfigServiceImpl.cfg.json
{
"app.name": "aem-demo",
"api.endpoint": "https://www.google.com",
"client.id": "8TfyfkUAB481AxOfn4UrqIyWIStKrTm8Jl",
"client.secret": "8TxmpkXAPRtLiOHIFHzjx4uKHlm1soAvO7I"
}
/system/console/configMgr
.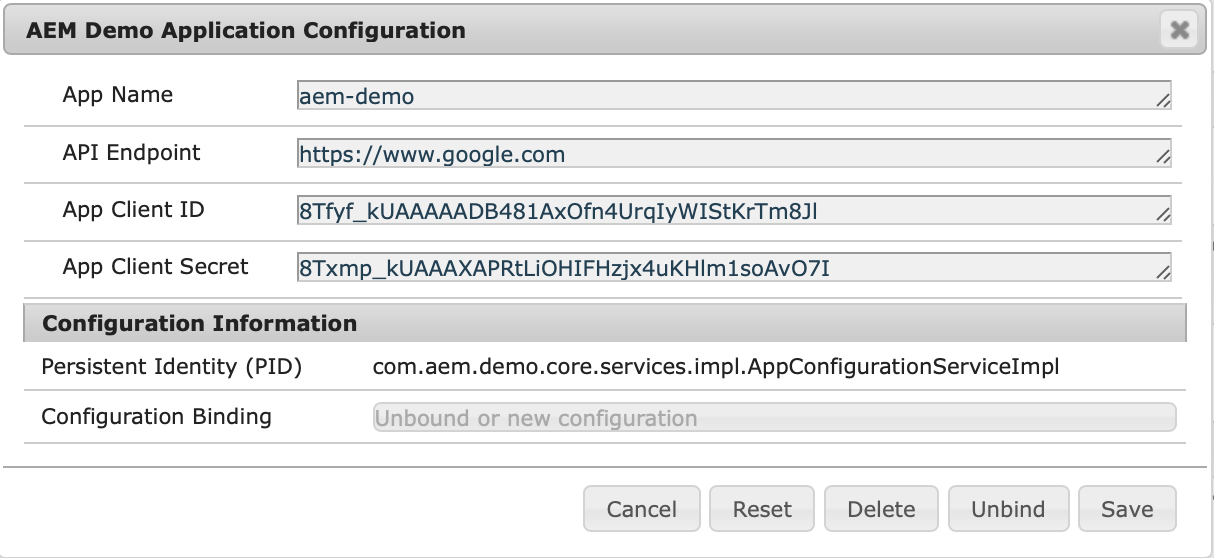
config / com.aem.demo.core.services.impl.AppConfigServiceImpl.cfg.json
{
"app.name": "aem-demo",
"api.endpoint": "$[env:API_ENDPOINT]",
"client.id": "$[secret:CLIENT_ID]",
"client.secret": "$[secret:CLIENT_SECRET]"
}
- Inline values: which are values that are hard-coded into the OSGi configuration and stored in Git, such as
app.name
. - Environment-specific values: which are values that vary between Development environments, and thus cannot be accurately targeted by run mode, particularly when multiple development environments are needed and share the same run mode.
- Secret values: which are values that must not be stored in Git for security reasons, such as
client.id
andclient.secret
.
/system/console/configMgr
is not accessible in AEM Cloud environment. To view configuration values, you can use Developer Console instead.